Atomic Design System in SwiftUI
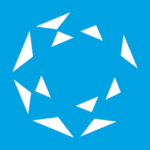
Introduction
Welcome to our deep dive into building modern UI design systems using SwiftUI and the principles of atomic design. In this blog post, we will explore the fundamentals of SwiftUI and how to leverage atomic design methodology to create scalable and maintainable design systems. Whether you are experienced or new to SwiftUI, this guide will provide you with valuable insights and practical examples to enhance your UI development skills in iOS.
SwiftUI is Apple's modern framework for building user interfaces across all Apple platforms. It uses a declarative syntax that allows developers to create stunning, app-wide user interfaces with minimal code.
Overview of What You'll Learn
- Understand the key features and benefits of SwiftUI.
- Learn the principles of atomic design and how they apply to UI development.
- Gain hands-on experience in creating an atomic design system with SwiftUI.
- Discover best practices for maintaining consistency and scalability in your design system.
- Explore theming and customization techniques to match brand guidelines.
- Explore practical code examples and step-by-step guides for building SwiftUI applications.
Introduction to SwiftUI
Overview of SwiftUI
SwiftUI is Apple's declarative framework for building user interfaces across all Apple platforms. Introduced in 2019, SwiftUI allows developers to create stunning and responsive UIs with minimal code by leveraging a modern, intuitive syntax. It was first introduced at WWDC 2019 and has since become an integral part of iOS, macOS, watchOS, and tvOS development.
What Are The Key Features and Benefits of SwiftUI?
- Declarative Syntax: SwiftUI uses a declarative syntax, making it easy to read and write. You simply describe what the UI should look like, and SwiftUI takes care of the rest.
- Cross-Platform Compatibility: Build UIs for iOS, macOS, watchOS, and tvOS using a single set of tools and APIs.
- Live Preview: Instantly see the results of your code changes with SwiftUI's live preview feature in Xcode.
- State Management: SwiftUI provides powerful state management tools to handle UI updates efficiently.
- Integration with Other Frameworks: SwiftUI seamlessly integrates with existing UIKit and AppKit components, allowing for gradual adoption in existing projects.
SwiftUI's Declarative Syntax and Its Facilitation of UI Development
SwiftUI employs a declarative programming paradigm, which contrasts with the imperative programming approach traditionally used in frameworks like UIKit. Declarative programming allows developers to specify what the user interface should do and look like in different states, without detailing the how of the control flow.
- Developers define the UI and its behavior by declaring the desired outcomes for different states. For instance, specifying that a welcome message should appear when logged in and a login button when logged out.
- SwiftUI handles the transitions between these states automatically, reducing the need for explicit state management and event handling code.
- This approach simplifies the development process by abstracting complex state management and allowing developers to focus on the UI's logic and appearance.
SwiftUI's declarative syntax also facilitates cross-platform development within the Apple ecosystem. It provides a unified framework for building applications across iOS, macOS, watchOS, and tvOS, eliminating the need to learn multiple frameworks for different platforms.
Despite its advantages, SwiftUI does not replace UIKit but rather builds on top of it, offering an additional layer of abstraction. While this abstraction simplifies development, it may limit customization options compared to imperative programming.
Why is SwiftUI Ideal for Building Modern UIs?
SwiftUI's modern approach to UI development aligns perfectly with the needs of today's developers. While its declarative nature simplifies the development process, its cross-platform capabilities ensure a consistent user experience across all Apple devices. By using SwiftUI, developers can create elegant, responsive, and scalable UIs with ease.
What is an Atomic Design System?
Explanation of Atomic Design Methodology
Atomic design is a methodology introduced by Brad Frost that breaks down the UI into five distinct stages: Atoms, Molecules, Organisms, Templates, and Pages. This approach encourages a systematic and hierarchical way of thinking about UI components, making it easier to build, maintain, and scale design systems.
Jetpack Compose
Check out our Atomic Design Systems in Jetpack Compose
Key Stages of Atomic Design and Their Importance for UI Consistency
Atomic Design, a methodology pioneered by Brad Frost, is a structured approach to creating design systems that ensure UI consistency. It is based on the concept of building complex interfaces from simple components, similar to how atoms form molecules in chemistry. The key stages of Atomic Design are:
- Atoms: These are the basic building blocks of the design system, including fonts, animations, and color palettes. Atoms are the foundational elements that inform everything else in the design system.
- Molecules: Molecules are combinations of atoms that create more complex UI elements, such as form labels or input fields. They serve as the backbone of the larger design system.
- Organisms: Organisms are groups of molecules that form distinct sections of a user interface, like headers or navigation bars. They define both the appearance and functionality of a mobile application.
- Templates: Templates are collections of organisms arranged at the page level. They provide a fixed context for the design system and help in visualizing the final layout of the website.
- Pages: Pages are specific instances of templates that represent the final product. They are crucial for testing the effectiveness of the design system and ensuring that the UI is ready for launch.
The importance of these stages lies in their ability to create a consistent and reusable design system. By breaking down the UI into smaller components, Atomic Design allows for a "mix and match" approach, enabling developers to reuse and repurpose elements efficiently. This results in straightforward layouts that are easier for developers to manage and for users to navigate, ultimately enhancing the overall user experience.
Importance of Design Systems and Atomic Design Principles
Design systems play a crucial role in modern UI development by providing a cohesive and reusable set of design elements and guidelines. Atomic design principles further enhance this approach by breaking down the UI into smaller, manageable components. This not only promotes consistency but also makes it easier to scale and maintain your design system over time.
Benefits of Using Atomic Design in Modern UI Development
Consistency: Ensures a unified look and feel across the entire application. Reusability: Promotes the creation of reusable components, reducing duplication and development time. Scalability: Facilitates the growth of the design system as the application evolves. Maintainability: Simplifies the process of updating and maintaining UI components.
Overview of the Five Stages: Atoms, Molecules, Organisms, Templates, and Pages
Atoms: The smallest building blocks of the UI, such as buttons, labels, and icons. Molecules: Combinations of atoms that form simple UI elements, like an input field with a label. Organisms: More complex components composed of multiple molecules and atoms, such as a header or a form. Templates: Page-level structures that define the layout and arrangement of organisms. Pages: Complete pages built using templates, representing the final UI.
Atomic Design in Mobile Applications
Example: Instagram Home Page Design
Creating the Atomic Design System
Design Tokens
Explanation of Design Tokens and Their Importance
Design tokens are a core aspect of design systems, representing the basic visual elements such as colors, typography, and spacing. They ensure consistency and enable easy updates across the entire UI by defining these elements in a centralized location.
Role of Design Tokens in Atomic Design
Design tokens play a crucial role in the Atomic Design methodology by serving as the smallest functional units that abstract visual and functional properties of design elements into reusable, modular pieces of code. These tokens include properties such as colors, typography, spacing, and breakpoints, which are essential for maintaining consistency across a design system.
Contribution to a Cohesive Design System in SwiftUI
- Consistency and Efficiency: Design tokens provide a centralized source of truth for all design elements, ensuring that designers and developers can access and use consistent design properties across different products and platforms. This is particularly beneficial in SwiftUI, where maintaining a cohesive look and feel is crucial.
- Flexibility and Scalability: By defining a shared language and set of standards for design properties, design tokens enable flexibility and scalability. This allows for quick creation of new elements and layouts, which is essential for the dynamic nature of SwiftUI applications.
- Ease of Maintenance: Design tokens allow for easy updates and maintenance. For instance, if a brand's color palette changes, updating the design token will automatically reflect these changes throughout the design system, saving time and reducing errors.
- Theme Customization: Design tokens facilitate the creation of different system themes, such as light and dark modes, by defining core design tokens that can be customized for various themes. This adaptability is vital for SwiftUI applications that need to support different user preferences or brand identities.
Overall, design tokens, when integrated with the Atomic Design methodology, contribute significantly to building robust and scalable design systems in SwiftUI by ensuring consistency, flexibility, and ease of maintenance.
Examples of Common Design Tokens
- Colors: Primary, secondary, background, text colors.
- Typography: Font families, sizes, weights.
- Spacing: Margins, padding, grid layout dimensions.
How to Implement Design Tokens in SwiftUI
In SwiftUI, you can implement design tokens using custom structs and extensions. Here's an example:
struct DesignTokens {
struct Colors {
static let primary = Color("PrimaryColor")
static let secondary = Color("SecondaryColor")
static let background = Color("BackgroundColor")
}
struct Typography {
static let titleFont = Font.system(size: 24, weight: .bold)
static let bodyFont = Font.system(size: 16, weight: .regular)
}
struct Spacing {
static let small: CGFloat = 8
static let medium: CGFloat = 16
static let large: CGFloat = 24
}
}
Atoms
Definition and Examples of Atomic Components in SwiftUI
Atoms are the basic building blocks of a UI. In SwiftUI, atoms include elements like buttons, texts, and icons.
Examples of SwiftUI's Declarative Syntax for Building Atomic Components
SwiftUI's declarative syntax allows developers to efficiently build atomic components by describing the UI elements and their behaviors in a straightforward manner. Here are some examples illustrating how this can be applied to create atomic components within an atomic design system:
Atoms
Atoms are the basic building blocks of a UI, such as buttons, texts, and icons. Below are examples of how to create these components using SwiftUI's declarative syntax:
struct PrimaryButton: View {
var title: String
var body: some View {
Button(action: {
// Button action
}) {
Text(title)
.font(DesignTokens.Typography.bodyFont)
.foregroundColor(.white)
.padding()
.background(DesignTokens.Colors.primary)
.cornerRadius(8)
}
}
}
PrimaryButton Component
struct PrimaryText: View {
var text: String
var body: some View {
Text(text)
.font(DesignTokens.Typography.titleFont)
.foregroundColor(DesignTokens.Colors.primary)
}
}
PrimaryText Component
struct Icon: View {
var systemName: String
var body: some View {
Image(systemName: systemName)
.resizable()
.aspectRatio(contentMode: .fit)
.frame(width: 24, height: 24)
.foregroundColor(DesignTokens.Colors.secondary)
}
}
Icon Component
Molecules
Explanation of How to Combine Atoms to Form Molecules
Molecules are combinations of atoms that form more complex UI elements. For example, an input field with a label can be considered a molecule.
Combining Atoms into Molecules in UI Design
The concept of combining atoms into molecules in UI design is a central aspect of the Atomic Design methodology, as introduced by Brad Frost. This approach enhances modularity by allowing designers to create simple, reusable components that serve as building blocks for more complex structures.
Enhancing Modularity
By combining atoms into molecules, designers adhere to the single responsibility principle, which encourages components to "do one thing and do it well." This approach simplifies testing, enhances reusability, and promotes consistency across the interface. Molecules provide a clear structure for creating more complex components, known as organisms, which are essential for building sophisticated UI systems.
Example: Search Form Molecule
An example of a molecule in action is a search form, which combines a label, input, and button. This molecule can be used wherever search functionality is needed, demonstrating how combining atoms into molecules creates modular, reusable components that enhance the overall design system.
// MARK: - Atoms
struct SearchLabel: View {
let text: String
var body: some View {
Text(text)
.font(.headline)
.foregroundColor(.primary)
}
}
struct SearchTextField: View {
@Binding var query: String
var placeholder: String
var body: some View {
TextField(placeholder, text: $query)
.padding(10)
.background(Color(.systemGray6))
.cornerRadius(8)
.overlay(
RoundedRectangle(cornerRadius: 8)
.stroke(Color.gray.opacity(0.5), lineWidth: 1)
)
}
}
struct SearchButton: View {
let action: () -> Void
var body: some View {
Button(action: action) {
Image(systemName: "magnifyingglass")
.padding()
.background(Color.blue)
.foregroundColor(.white)
.clipShape(Circle())
}
}
}
// MARK: - Molecule
struct SearchForm: View {
@State private var searchQuery: String = ""
var onSearch: (String) -> Void
var body: some View {
HStack {
SearchLabel(text: "Search")
SearchTextField(query: $searchQuery, placeholder: "Enter text...")
SearchButton {
onSearch(searchQuery)
}
}
.padding()
}
}
How the atoms (building blocs) should look like and how they are used to create the molecule component
Organisms
Creating Complex Components by Combining Molecules and Atoms
Organisms are larger components that consist of multiple molecules and atoms. These components form significant parts of the UI, such as a login form or a navigation bar.
Code Examples and Best Practices
struct LoginForm: View {
@State private var username: String = ""
@State private var password: String = ""
var body: some View {
VStack(spacing: DesignTokens.Spacing.large) {
LabeledInputField(label: "Username", text: $username)
LabeledInputField(label: "Password", text: $password)
PrimaryButton(title: "Login")
}
.padding(DesignTokens.Spacing.large)
.background(DesignTokens.Colors.background)
.cornerRadius(8)
}
}
Templates
Designing the Structure of a Page by Combining Organisms
Templates define the overall layout of a page by arranging organisms in a structured manner.
Code Examples and Considerations for Reusable Templates
struct MainLayout<Content: View>: View {
let content: Content
var body: some View {
VStack {
NavigationBar()
content
.padding(DesignTokens.Spacing.medium)
Spacer()
}
.background(DesignTokens.Colors.background)
.edgesIgnoringSafeArea(.all)
}
}
struct NavigationBar: View {
var body: some View {
HStack {
Icon(systemName: "arrow.left")
Spacer()
PrimaryText(text: "Title")
Spacer()
Icon(systemName: "gear")
}
.padding()
.background(DesignTokens.Colors.primary)
}
}
Pages
Assembling Templates to Form Complete Pages
Pages are the final UI screens that users interact with, created by combining templates and filling them with content.
Examples of Final Pages and How They Utilize the Design System Components
struct LoginPage: View {
var body: some View {
MainLayout {
LoginForm()
}
}
}
struct ContentView: View {
var body: some View {
NavigationView {
LoginPage()
.navigationBarHidden(true)
}
}
}
Best Practices and Tips
Consistency and Scalability
Tips for Maintaining Consistency Across the Design System
- Use Design Tokens: Centralize your design tokens to ensure consistency across the UI.
- Component Libraries: Build and maintain a library of reusable components.
- Naming Conventions: Follow consistent naming conventions for components and styles.
Strategies for Scaling the Design System as the Project Grows
- Modular Design: Break down components into smaller, reusable modules.
- Documentation: Maintain thorough documentation of your design system.
- Regular Reviews: Periodically review and update your design system to accommodate new requirements.
How Atomic Design Contributes to Scalability and Adaptability
Atomic Design is a methodology that enhances the scalability and adaptability of design systems as applications grow. This approach, introduced by Brad Frost, breaks down complex user interfaces into smaller, manageable components, facilitating a modular and reusable design structure.
Scalability
- Modular Components: Atomic Design's structure, consisting of atoms, molecules, organisms, templates, and pages, allows for the creation of a library of reusable design elements. This modularity ensures that as an application grows, new designs can be developed quickly by reusing existing components, rather than starting from scratch.
- Consistency: By maintaining a consistent set of design elements, Atomic Design ensures that new features or pages adhere to the established design language, supporting a cohesive brand identity across the application.
Adaptability
- Flexible Design System: The hierarchical nature of Atomic Design allows for easy updates and modifications. As the application evolves, components can be adjusted or replaced without disrupting the entire system, ensuring that the design system remains relevant and up-to-date.
- Efficient Collaboration: A shared design system facilitates better collaboration among design teams, as standardized components reduce the time and effort needed to create new designs, allowing teams to adapt quickly to changing requirements.
Conclusion
Overall, Atomic Design provides a structured approach to building scalable and adaptable design systems. By leveraging its modular framework, designers can efficiently manage growing applications while maintaining consistency and flexibility in their design processes.
Theming and Customization
Implementing Themes in SwiftUI
SwiftUI allows you to implement themes by using environment values and custom modifiers.
struct CustomTheme {
static let light = CustomTheme(
primaryColor: .blue,
secondaryColor: .gray,
backgroundColor: .white,
textColor: .black
)
static let dark = CustomTheme(
primaryColor: .white,
secondaryColor: .gray,
backgroundColor: .black,
textColor: .white
)
let primaryColor: Color
let secondaryColor: Color
let backgroundColor: Color
let textColor: Color
}
struct ThemedViewModifier: ViewModifier {
let theme: CustomTheme
func body(content: Content) -> some View {
content
.environment(\.theme, theme)
}
}
extension EnvironmentValues {
var theme: CustomTheme {
get { self[CustomThemeKey.self] }
set { self[CustomThemeKey.self] = newValue }
}
}
struct CustomThemeKey: EnvironmentKey {
static let defaultValue: CustomTheme = .light
}
Conclusion
In this blog post, we have explored the fundamentals of building modern UI design systems using SwiftUI and atomic design principles. We have covered the basics of SwiftUI and atomic design methodology in order to create and implement an atomic design system with simple code examples.
We encourage you to start building your own design using the principles and techniques discussed in this article. Experiment, iterate, and refine your components to create a cohesive and scalable design system that meets your project's needs.
Frequently Asked Questions
How does SwiftUI's declarative syntax benefit UI development?
SwiftUI's declarative syntax allows developers to specify what the UI should do and look like in different states without detailing how to manage the control flow. This simplifies the development process by abstracting complex state management and allowing developers to focus on the UI's logic and appearance.
What are the key stages of Atomic Design?
The key stages of Atomic Design are Atoms, Molecules, Organisms, Templates, and Pages. These stages help create a consistent and reusable design system by breaking down the UI into smaller components.
Why are design tokens important in Atomic Design?
Design tokens are crucial in Atomic Design as they serve as the smallest functional units that abstract visual and functional properties of design elements into reusable, modular pieces of code. They ensure consistency, flexibility, and ease of maintenance across a design system.
How does Atomic Design contribute to scalability and adaptability?
Atomic Design enhances scalability and adaptability by breaking down complex user interfaces into smaller, manageable components. This modular and reusable design structure allows for quick development of new designs and easy updates, ensuring the design system remains relevant and up-to-date.
Further Reading and Resources
Links to Additional Resources, Documentation, and Tutorials
Suggestions for Books, Articles, or Courses on Atomic Design and SwiftUI
- Books: "SwiftUI for Absolute Beginners" by Jayant Varma, "Atomic Design" by Brad Frost.
- Articles: "Building Design Systems with SwiftUI" on Medium.
- Courses: "SwiftUI Masterclass" on Udemy, "Building iOS Apps with SwiftUI" on Coursera.