Debug GPU Overdraw: How to Improve Android UI Performance
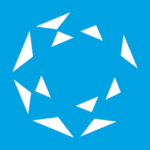
Android UI Performance: GPU Overdraw
Introduction
Ensuring optimal performance in Android applications is crucial for providing a smooth user experience and preserving device battery life. In this post, we will go into two significant aspects of UI performance: overdraw and rendering. We'll explore what they are, how to debug and analyze them, and provide real-life examples and strategies for improvement. We'll also cover how to use the Android Studio Profiler to diagnose and optimize performance issues related to CPU, memory, and energy usage.
UI Performance Overdraw
What is an overdraw?
Overdraw occurs when your app draws the same pixel more than once within the same frame. This is a common performance issue because, in most cases, pixels that are overdrawn do not end up contributing to the final rendered image.
Why is Android GPU overdraw important for app performance?
This overdraw can lead to performance issues because the GPU is required to perform extra rendering work for pixels that are not visible to the user.
Importance of Managing GPU Overdraw
Why is Visualizing GPU Overdraw Important?
Visualizing GPU overdraw is crucial for several reasons:
Performance Optimization: By identifying areas with excessive overdraw, developers can pinpoint inefficiencies. High overdraw can lead to increased battery consumption and reduced performance, causing apps to run slower and devices to overheat. Reducing overdraw is crucial for optimizing app performance, as it minimizes unnecessary GPU workload, leading to smoother UI rendering.
Rendering Efficiency: Visualizing overdraw helps developers understand where they can minimize unnecessary rendering. This leads to smoother animations and faster load times, enhancing the overall user experience. By decreasing overdraw, apps can use system resources more efficiently, which is particularly important for devices with less powerful GPUs.
Resource Management: Understanding and managing overdraw ensures better utilization of GPU resources, allowing more complex and visually appealing applications without compromising performance.
Improved User Experience: Lower overdraw levels contribute to a more responsive and fluid user interface, enhancing the overall user experience.
By employing visualization tools that color-code elements based on their rendering frequency, developers can easily spot problem areas and optimize their applications. This proactive approach is key to maintaining robust and efficient mobile applications in a competitive app market.
Read our other articles on Android performance
UI Performance Rendering
The rendering process relies on multiple system parts, and each task might lead to rendering issues that can point to the necessary optimizations.
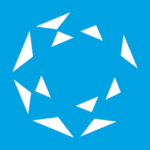
Atomic Design System in Jetpack Compose
Our guide on creating an atomic design system using Jetpack Compose
In other terms, an application might be doing more rendering work than necessary, which can be a performance problem due to the extra GPU effort to render pixels that won’t be visible to the user.
Fixing overdraw involves using available on-device tools, like showing the GPU Overdraw, and then adjusting your view hierarchy to reduce areas where it may be occurring. On your Android device, simply go to the Developer options screen and turn on the “Show GPU Overdraw” flag.
Enabling this option will let you know when and where the overlay is happening so you can judge if it’s affecting performance.
Analyzing an overdraw
Once you enable this flag, Android shows overdraw amounts on your screen by tinting pixels in different colors. If you’ve only rendered a pixel one time, you should see it in its true color with no tint.
However, as the overdraw increases, so do the colors. 1x overdraw, for example, is tinted blue, meaning that you’ve now redrawn this pixel one extra time. 2x, 3x, and 4x overdraw follow the same pattern.
Interpreting the Debug GPU Overdraw Output: A Color Guide The color key for Debug GPU Overdraw output is essential for understanding the level of overdraw affecting each pixel on your screen. Here's a breakdown of what each color signifies in this context:
True Color: This indicates that no overdraw is occurring, ensuring optimal performance as each pixel is drawn only once.
Blue: A blue hue signifies that a pixel is drawn twice, introducing some overdraw that could impact performance slightly.
**Green: **When you see green, it means the pixel is overdrawn three times, suggesting moderate performance concerns that may need attention.
**Pink: **A pink color indicates that the pixel is drawn four times, which could significantly affect performance and warrants investigation for optimization.
Red: Red signifies a severe level of overdraw, with pixels being drawn five or more times. This level of overdraw can severely impact performance, necessitating immediate corrective action.
The goal of your application is to reduce as much overdraw as possible, moving from lots of deep red to more blues.
Real-life examples
Now that we know what GPU Overdraw means, let’s take a look at some popular apps and see how they perform.
As you can see from these screenshots, very few apps take into consideration overdraw optimization. While it doesn’t directly affect the application’s performance, it does increase battery usage due to additional GPU rendering effort.
What are the consequences of GPU overdraw?
Apps that suffer from GPU overdraw, like the apps above mentioned, can face several consequences:
- Performance Degradation: Excessive overdraw can lead to slower app performance as the GPU works harder to render the same frame multiple times.
- Increased Battery Consumption: Higher GPU activity can drain the device's battery more quickly, reducing the overall battery life.
- Thermal Issues: Continuous high GPU usage can cause the device to heat up, potentially leading to thermal throttling and further performance issues.
Fixing an overdraw
Fixing an overdraw heavily depends on the complexity of the application’s screens. However, several strategies can help reduce or eliminate overdraw. Let's look at the most common fix: removing unneeded backgrounds in layouts.
Removing unneeded backgrounds in layouts
Eliminate backgrounds that are not visible to the user, especially when they are completely covered by other elements. Use the Layout Inspector tool to identify and remove such backgrounds. Set the window background to the main app color and avoid defining background values for containers above it.
Here's an example of views being overdrawn. All the red areas in the image suggest that the views are redrawn on top of each other. Let's look at the code and figure out what's wrong.
Here we have a parent RelativeLayout with a white background, a child LinearLayout also with a white background, and another child TextView with a white background.
Now it is very important that you notice that the white color is being used multiple times in the code. First the RelativeLayout is drawn with the white background, then the child LinearLayout also draws a white color, and the TextView draws white again.
Therefore, we are wasting GPU cycles here by redrawing on the same pixels multiple times. Note that this is not because the colors are same, but because we are redrawing the pixels again and again.
We now know that the color white is being drawn multiple times on the same view. All we have to do is to remove the white background from the LinearLayout and the TextView.
After changes in the code the overdraw is much lesser, we can now see that there are no more red marks, meaning that there is no heavy overdraw happening anymore.
Other practices
There are many other common practices that one can follow to reduce overdraw, some of them are:
- Removing invisible views. That is, removing views that are completely covered by other views.
- Reducing the use of transparency. For example, instead of using transparency to create a color, just find that color. Instead of using transparency to create a shine-through effect for two overlapping images, preprocess the two images into one. Minimize the use of transparent pixels, as they require existing pixels to be drawn first for proper blending. Consider alternatives, such as using gray text instead of black text with a translucent alpha value, to achieve similar visual effects with better performance. Watch the video Hidden Costs of Transparency for more insights.
- Flattening and reorganizing the view hierarchy to reduce the number of views. Optimize the view hierarchy to reduce overlapping UI objects. This minimizes the drawing of both seen and unseen pixels, improving performance. For more guidance, refer to Performance and view hierarchies.
- Resizing or rearranging the views so that they do not overlap.
Understanding GPU Overdraw: Benefits in App Development
Enhance Performance Recognizing GPU overdraw is crucial for optimizing an app’s performance. By pinpointing areas where rendering is excessive, developers can streamline processes, reducing the workload on the device’s graphics processor. This leads to smoother animations and a more responsive user interface, providing a better experience for users across various devices.
Improve Battery Life
Excessive rendering not only slows down performance but also drains the battery faster. By minimizing overdraw, developers can ensure their app is more energy-efficient. This optimization helps extend battery life, which is a significant concern for users, especially those who frequently use resource-intensive applications.
Reduce Excess Resource Usage
An app that efficiently manages GPU overdraw avoids unnecessary use of computational power and system resources. This not only improves the app’s overall footprint but also allows it to run more efficiently alongside other applications. The reduction in resource consumption can be critical in environments where device capabilities are limited, ensuring broader compatibility and performance consistency.
Enhance User Experience
Ultimately, understanding and addressing GPU overdraw contributes to a seamless user experience. By reducing lag and preventing visual stutters, apps can deliver a polished, professional look and feel. Users are more likely to engage with and recommend applications that run smoothly and look appealing, driving greater satisfaction and retention.
Streamline Development and Debugging
Early identification of overdraw issues can simplify the development and debugging process. Developers can more easily focus their efforts on optimizing problematic areas, speeding up development cycles. This proactive approach can prevent potential performance issues from arising post-launch, saving time and effort in the long run.
In summary, understanding GPU overdraw is essential for app developers aiming to boost performance, improve battery efficiency, and enhance the overall user experience.
Related Articles
Building Offline Apps: A Fullstack Approach to Mobile Resilience
A step-by-step guide to building offline mobile apps
Atomic Design System in SwiftUI
We will explore the fundamentals of SwiftUI and how to leverage atomic design methodology to create scalable and maintainable design systems.